Depth Bias
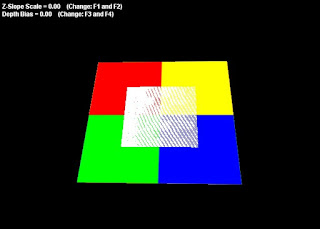
To solve Z fighting issue: use depth bias. An application can help ensure that coplanar polygons are rendered properly by adding a bias to the z-values that the system uses when rendering the sets of coplanar polygons. To add a z-bias to a set of polygons, call the IDirect3DDevice9::SetRenderState method just before rendering them, setting the State parameter to D3DRS_DEPTHBIAS, and the Value parameter to a value between 0-16 inclusive. A higher z-bias value increases the likelihood that the polygons you render will be visible when displayed with other coplanar polygons. Offset = m * D3DRS_SLOPESCALEDEPTHBIAS + D3DRS_DEPTHBIAS where m is the maximum depth slope of the triangle being rendered. m = max(abs(delta z / delta x), abs(delta z / delta y)) reference: http://msdn.microsoft.com/en-us/library/bb205599%28VS.85%29.aspx Z Slope Scale = 0.0, Depth Bias = 0.0